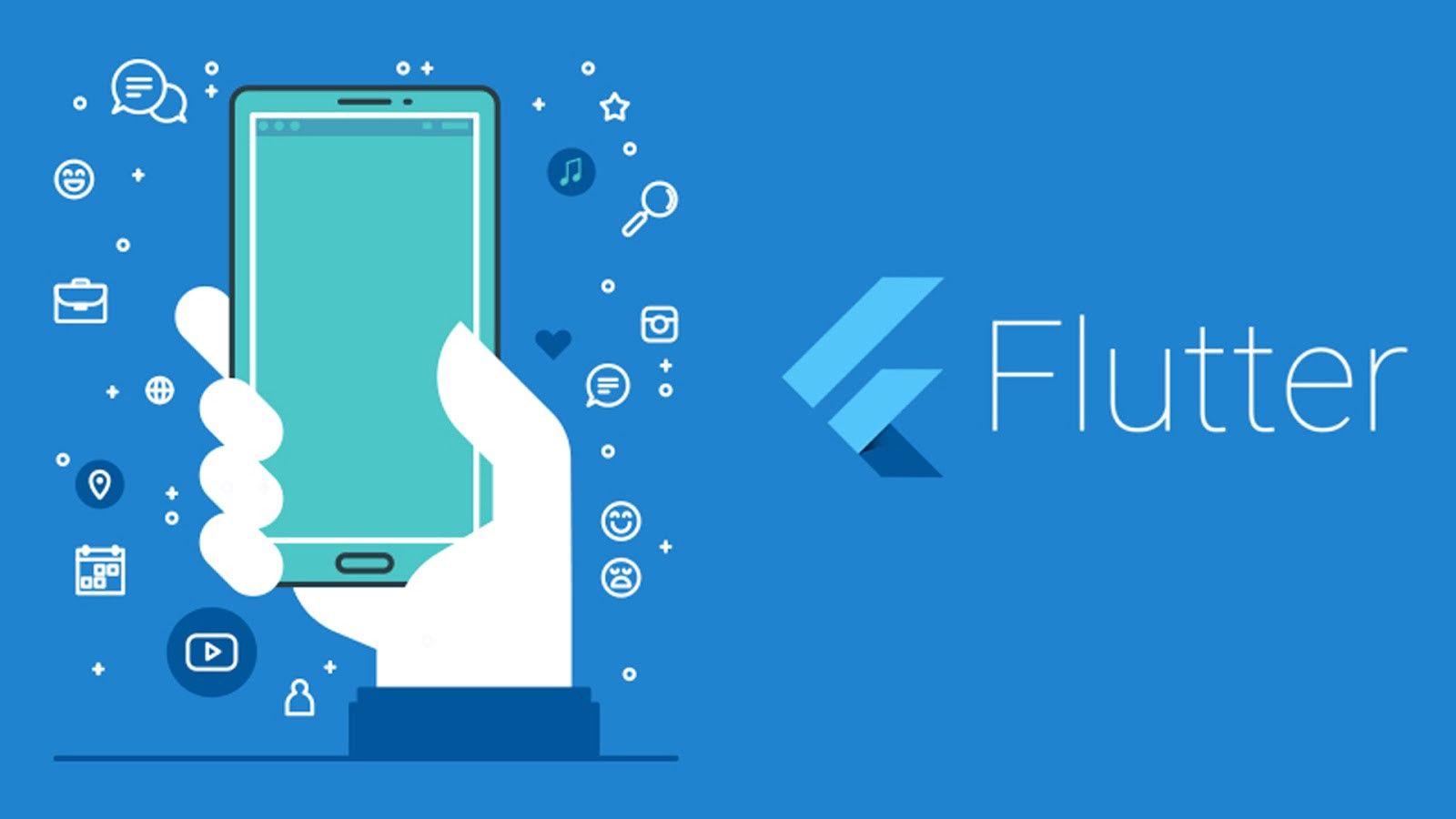
Introduction:
Taking photos is a common requirement in many mobile applications. However, in some cases, you may need to take multiple photos at once, for example, when creating a photo album or when capturing multiple images for an image recognition task. In this tutorial, we will create a Flutter package that allows you to take multiple photos from the camera at once.
Prerequisites:
Before we get started, make sure that you have the following installed:
Flutter SDK
Android Studio or VS Code
An Android or iOS device for testing
Step 1: Create a new Flutter package Open Android Studio or VS Code and create a new Flutter package. You can do this by selecting File > New > Flutter Package. Give your package a name and select the location where you want to store it.
You can find this Package here:
https://pub.dev/packages/multiple_image_camera
Step 2: Add dependencies
In your pubspec.yaml file, add the following dependencies:
multiple_image_camera: ^0.0.1
You can Also Install with the command :
flutter pub add multiple_image_camera
Import it for use :
import 'package:multiple_image_camera/multiple_image_camera.dart';
Step 3:
For iOS
The multiple_image_camera plugin compiles for any version of iOS, but its functionality requires iOS 10 or higher. If compiling for iOS 9, make sure to programmatically check the version of iOS running on the device before using any multiple_image_camera plugin features. The device_info_plus plugin, for example, can be used to check the iOS version. Add two rows to the ios/Runner/Info.plist:
one with the key Privacy — Camera Usage Description and a usage description. and one with the key Privacy — Microphone Usage Description and a usage description. If editing Info.plist as text, add:
NSCameraUsageDescription your usage description here
NSMicrophoneUsageDescription your usage description here
For Android
Change the minimum Android sdk version to 21 (or higher) in your android/app/build.gradle file.
minSdkVersion 21
Features:
- Display live camera preview in a widget.
- You can Switch Camera if you want to take photo from front Camera.
- Take Multiple Photos at once and them in the list.
- This package contains pre-canned animations for commonly-desired effects.
- This package also have Camera shutter vibration.
- Ability for Show images that are clicked and added to the list.
- You can zoom in or zoom out with pinch to zoom.
Screenshots and Video :
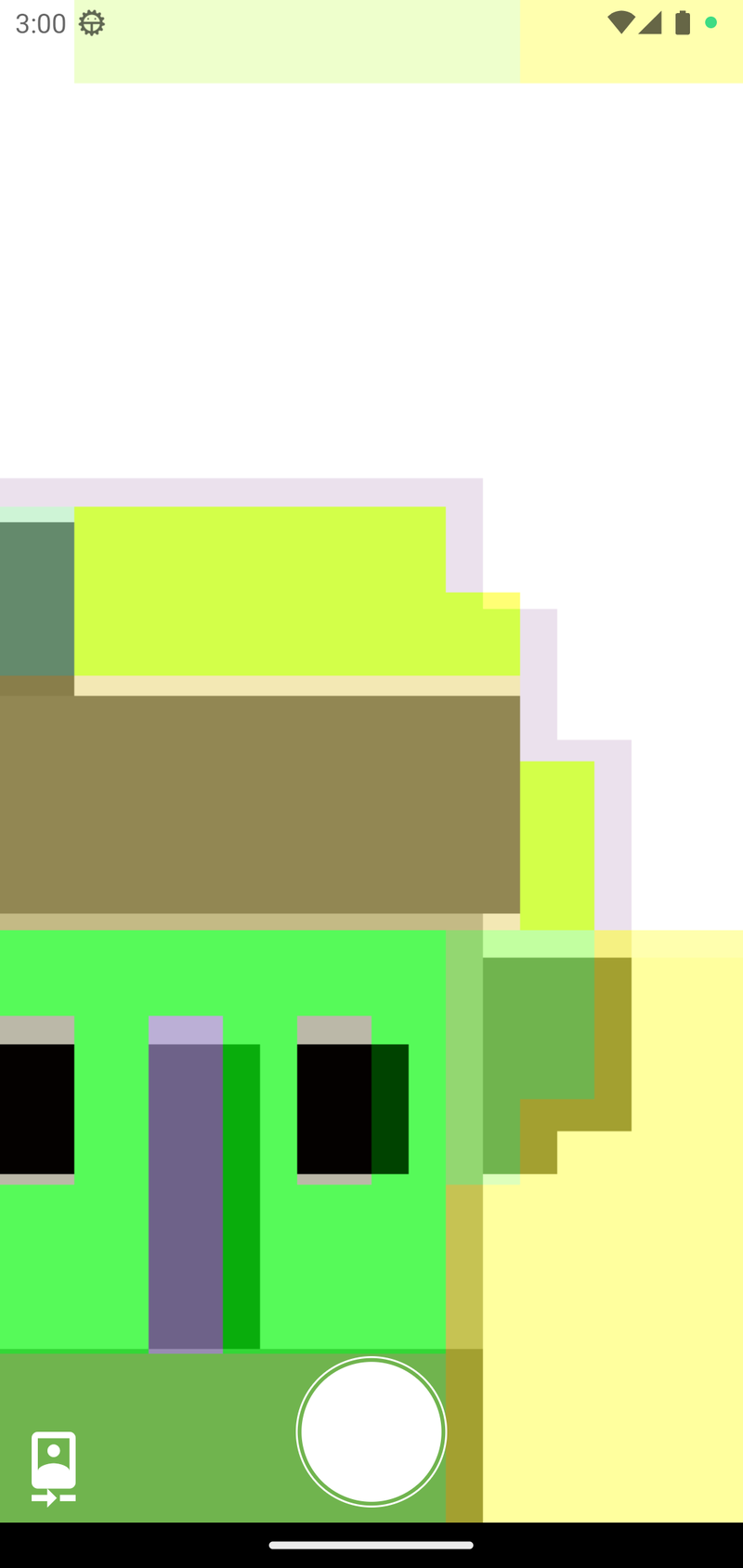
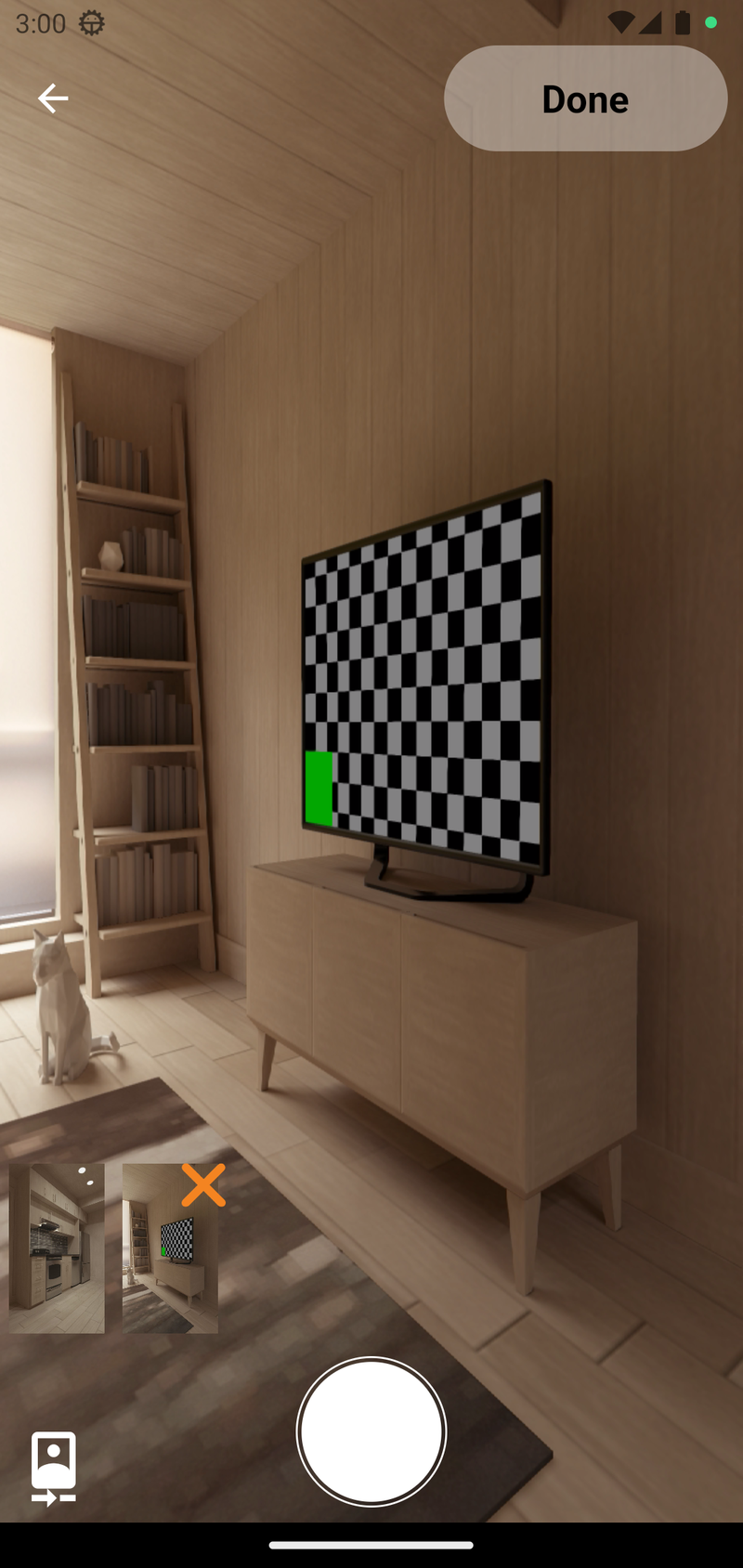
Usage:
Here is a small example flutter app displaying a full screen camera preview with taking multiple images.
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:multiple_image_camera/multiple_image_camera.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
List<MediaModel> imageList = <MediaModel>[];
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Multiple Image Camera',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(
imageList: imageList,
),
);
}
}
class MyHomePage extends StatefulWidget {
final List<MediaModel> imageList;
const MyHomePage({super.key, required this.imageList});
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
List<MediaModel> images = [];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(),
body: Column(
children: [
ElevatedButton(
child: const Text("Capture"),
onPressed: () async {
MultipleImageCamera.capture(context: context).then((value) {
setState(() {
images = value;
});
});
},
),
Expanded(
child: ListView.builder(
shrinkWrap: true,
itemCount: images.length,
itemBuilder: (context, index) {
return Image.file(File(images[index].file.path));
}),
)
],
),
);
}
}
Conclusion:
In conclusion, taking multiple photos from a camera at once can be a great way to capture a series of moments or different angles in a short amount of time. By using burst mode or continuous shooting mode on your camera, you can capture a series of images in rapid succession. Overall, taking multiple photos at once can be a valuable package for any flutter developer looking to capture a range of images quickly and efficiently.
Outro:
Thank you for taking the time to read my blog. I hope you found the information and insights shared here to be informative, thought-provoking, and useful. Remember, knowledge is power, and the more we learn, the better equipped we are to make informed decisions and navigate the complexities of our world. So keep seeking knowledge, asking questions, and exploring new ideas. And most importantly, don’t forget to apply what you’ve learned to make a positive impact in your life and the lives of those around you. Thanks again for reading!