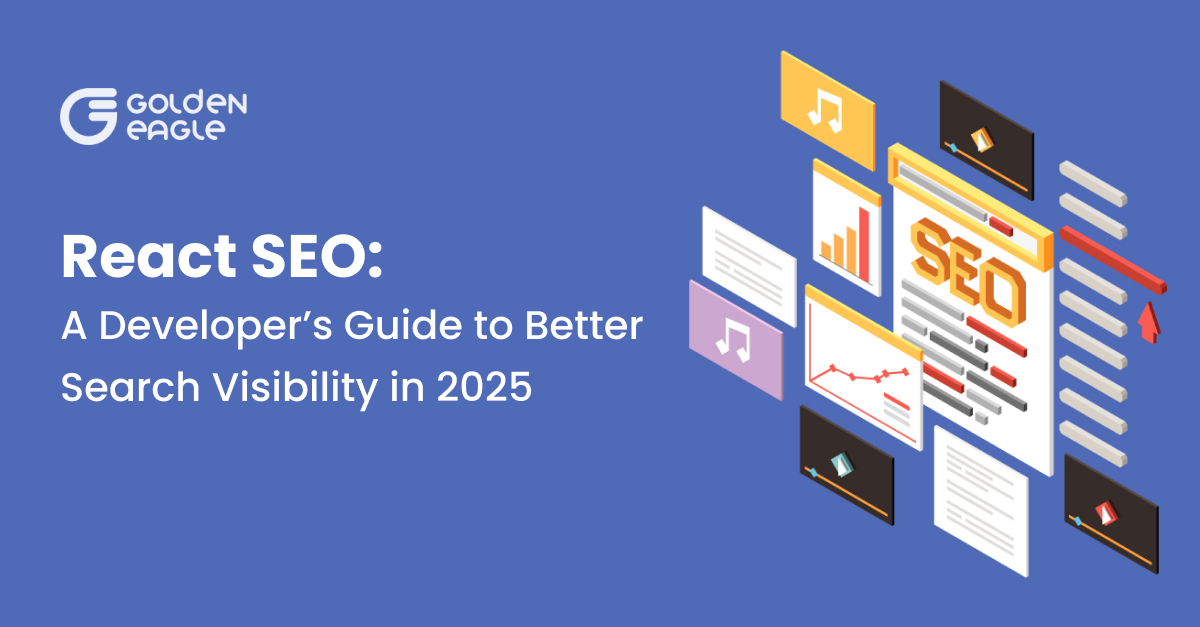
React is one of the most popular JavaScript libraries for building web applications, but it comes with a challenge—SEO optimization. Since React apps often rely on Client-Side Rendering (CSR), search engines struggle to crawl and index their content, leading to poor rankings.
This guide will help you understand the SEO challenges in React apps and provide proven techniques to improve your app’s search visibility. Whether you choose Server-Side Rendering (SSR), Static Site Generation (SSG), or pre-rendering, these strategies will make your React app more search-engine-friendly.
We’ll cover:
The SEO limitations of React SPAs
Solutions like Next.js, React Helmet, pre-rendering, and structured data
Performance optimization for better search rankings
Tools to monitor and improve your SEO
Let’s dive in!
1. Understanding SEO Challenges in React
How Search Engines Crawl Websites
Search engines like Google, Bing, and Yahoo crawl and index pages by reading HTML content. Traditional server-rendered websites provide fully-formed HTML, making it easy for search engines to index them.
However, React apps (SPAs) primarily use JavaScript to dynamically render content, which creates a problem:
- Search engines may not execute JavaScript properly, resulting in blank pages in search results.
- The page content is loaded after the initial HTML response, making it invisible to crawlers.
CSR vs. SSR vs. SSG: Which is Best for SEO?
- Client-Side Rendering (CSR)
- Poor SEO impact
- Content loads dynamically via JavaScript, making it hard for crawlers to index.
- Server-Side Rendering (SSR)
- Good SEO impact
- HTML is generated on the server and sent to the client, ensuring search engines receive fully-formed content.
- Static Site Generation (SSG)
- Best SEO impact
- Pre-renders HTML at build time for fast performance and SEO-friendly pages. Ideal for blogs and landing pages.
Solution: Use SSR or SSG with frameworks like Next.js for better SEO.
2. Solutions for Better SEO in React Apps
A. Use Next.js for Server-Side Rendering (SSR) and Static Site Generation (SSG)
Next.js is the best framework for SEO-friendly React apps. It allows SSR and SSG, ensuring search engines receive fully rendered pages.
Example: Implementing SSR in Next.js
import { GetServerSideProps } from 'next';
export default function Home({ data }) {
return (
<div>
<h1>{data.title}</h1>
<p>{data.description}</p>
</div>
);
}
export const getServerSideProps: GetServerSideProps = async () => {
const data = { title: "React SEO Guide", description: "Improve SEO in your React apps" };
return { props: { data } };
};
💡 For static pages, use SSG instead of SSR for better performance.
B. Using React Helmet for Meta Tags
Meta tags improve search rankings and social media previews. Use React Helmet Async to dynamically update meta tags.
Example: Adding Meta Tags
import { Helmet } from "react-helmet-async";
const HomePage = () => {
return (
<div>
<Helmet>
<title>Best React SEO Guide</title>
<meta name="description" content="Learn how to improve SEO in React apps" />
<meta property="og:title" content="React SEO Tips" />
<meta property="og:description" content="Boost your React app’s SEO with these techniques." />
</Helmet>
<h1>Welcome to My SEO-Optimized Page</h1>
</div>
);
};
export default HomePage;
C. Using Pre-Rendering for CSR Apps
If SSR or SSG is not an option, use pre-rendering services like Prerender.io to generate static HTML pages for search engines.
D. Improving Page Load Speed for SEO
Page speed is a Google ranking factor. Optimize React apps with:
✅ Lazy Loading images using next/image.
✅ Reducing JavaScript bundle size using React.lazy().
✅ Using a CDN for static assets.
Example: Lazy Loading in React
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<React.Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</React.Suspense>
);
}
E. Optimizing URLs and Routing
Clean URLs improve SEO rankings. Use Next.js dynamic routes for structured URLs.
Example: SEO-Friendly URLs in Next.js
/ pages/blog/[slug].tsx
import { useRouter } from 'next/router';
const BlogPost = () => {
const router = useRouter();
const { slug } = router.query;
return <h1>Blog Post: {slug}</h1>;
};
export default BlogPost;
F. Implementing Structured Data (Schema Markup)
Structured data helps Google understand your content and enables rich search results.
Example: Adding JSON-LD Schema
import { Helmet } from "react-helmet-async";
const StructuredData = () => {
return (
<Helmet>
<script type="application/ld+json">
{JSON.stringify({
"@context": "https://schema.org",
"@type": "Article",
"headline": "Improving SEO in React Apps",
"author": "John Doe",
})}
</script>
</Helmet>
);
};
export default StructuredData;
3. Testing and Monitoring SEO Performance
Use these tools to track SEO progress:
🔹 Google Search Console – Monitor indexing & fix issues.
🔹Google Lighthouse – Test speed & SEO score.
🔹Screaming Frog – Crawl your site for SEO insights.
Conclusion
Optimizing SEO in React apps requires SSR, SSG, metadata optimization, structured data, and page speed improvements. By implementing these techniques, you can boost your React app’s visibility and improve search rankings.
🚀 Ready to optimize your React app? Start implementing these SEO techniques today!
Frequently Asked Questions (FAQs) on React SEO
Does Google index JavaScript-heavy React apps?
Yes, but with limitations. While Googlebot can execute JavaScript, it processes React apps in two waves (initial HTML first, JavaScript execution later). This delay can cause indexing issues. Using Server-Side Rendering (SSR) or Static Site Generation (SSG) improves indexing. Additionally, using Google Search Console’s URL Inspection tool helps check how Google views your React pages.
How does Core Web Vitals affect React SEO?
Core Web Vitals measure page experience, affecting search rankings. Optimizing LCP, FID, and CLS improves SEO.
Can I use React with WordPress for SEO benefits?
Yes! Using React with a headless WordPress setup allows dynamic content while maintaining SEO benefits from WordPress’s backend.